SUM OF SERIES
CALCULATE THE SUM OF SERIES OF NUMBERS WITH CARRY
THEORY :
In 8085 microprocessor, In this program, we perform addition for 'n' numbers. Where we define the total no's to be added. All the values will be added with the accumulator. And this problem we have a new carry flag to enable the carry where we can see 16-bit resultant value.
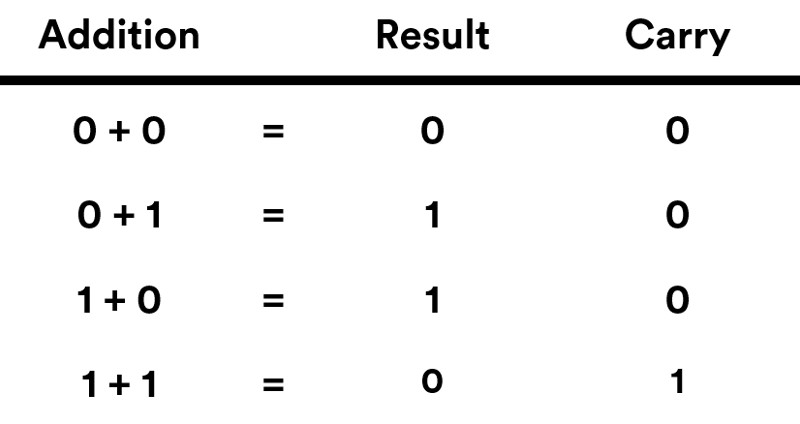
ALGORITHM :
Step 1: LXI H,2000h; to load the address in H-L register pair using LXI H from 16-bit location 2000h.
Step 2: MOV C, M; to move the value of 2000h memory address value into the C register.
Step 3: XRA A; to perform XOR operation.
Step 4: MOV D, A; to move the accumulator value to D register.
Step 5: INX H; to increase the value of the H-L register pair to get the next memory location.
Step 6: ADD M; to add memory location value with accumulator.
Step 7: JNC ; Jump on non-carry on INR D step 8.
Step 8: INR D; Increment register by 1 for D register.
Step 9: DCR C; to decrement the value of C register.
Step 10: JNZ; to jump on non zero to INX H.
Step 11: INX H; to increment H-L pair.
Step 12: MOV M, D; to store D register value in a memory location which is 2004h.
Step 13: INX H; to increment H-L register pair.
Step 14: MOV M, A; to move the value of Accumulator to memory.
Step 15: HLT; to stop the program.

IMPLEMENTATION :
Location | Mnemonics | Hex Code |
---|---|---|
3000 | LXI H | 21 |
3001 | 00 | 00 |
3002 | 20 | 20 |
3003 | MOV C, M | 4E |
3004 | XRA A | AF |
3005 | MOV D, A | 47 |
3006 | INX H | 23 |
3007 | ADD M | 86 |
3008 | JNC | D2 |
3009 | 0B | 0B |
300A | 30 | 30 |
300B | INR D | 14 |
300C | DCR C | 0D |
300D | JNZ | C2 |
300E | 06 | 06 |
300F | 30 | 30 |
3010 | INX H | 23 |
3011 | MOV M, D | 72 |
3012 | INX H | 23 |
3013 | MOV M, A | 77 |
3014 | HLT | 76 |
C++ PROGRAM:
#include <iostream>
using namespace std;
int main()
{
int arr[100];
int i, n, sum=0;
cout<<"Enter size of the array: ";
cin>>n;
cout<<"Enter" << n << "elements in the array: ";
for(i=0; i<n; i++)
{
cin>>arr[i];
}
for(i=0; i<n; i++)
{
sum += arr[i];
}
cout<<"Sum of all elements of array = "<<sum;
return 0;
}
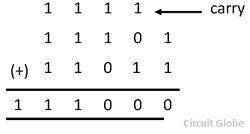
INPUT AND OUTPUT :
Location | Input |
---|---|
2000 | 02 |
2001 | 0F |
2002 | 0F |
Location | Output |
---|---|
2003 | 02 |
2004 | 1E |
DISCUSSION :
In this program, we tried to solve the problem with repetitive addition with a loop. where we predefine that loop iteration times and given values will add with accumulator. we also tried to show and store the carrying value. And also exampled value is also given as 0Fh + 0Fh and the carry of that 02h and the summation value is 1Eh.
YOU MIGHT LIKE:
FAQ:
1> Which language is used in microcontroller?
Ans: Microcontrollers were originally programmed only in assembly language, but various high-level programming languages, such as C, Python and JavaScript, are now also in common use to target microcontrollers and embedded systems.
2> What are the three types of language processor?
Ans: There are three types of language processors:
- Assembler.
- Interpreter.
- Compiler.
3> What is difference between microprocessor and micro controller?
Ans: Microprocessor consists of only a Central Processing Unit, whereas Micro Controller contains a CPU, Memory, I/O all integrated into one chip. The microprocessor uses an external bus to interface to RAM, ROM, and other peripherals, on the other hand, Microcontroller uses an internal controlling bus.
4> Is Raspberry Pi a microcontroller?
Ans: An Arduino is a microcontroller motherboard. A microcontroller is a simple computer that can run one program at a time, over and over again. It is very easy to use. A Raspberry Pi is a general-purpose computer, usually with a Linux operating system, and the ability to run multiple programs.
5> What are the 3 most important parts of a microprocessor?
Ans: There are three most important Microprocessor Components, i.e.
CPU.
Bus.
Memory.

6> What is the operation of add B instruction in 8085 MP?
Ans: There are some of the important instructions in 8085 microprocessor. 1. ADD: - The content of operand are added to the content of the accumulator and the result is stored in Accumulator. Eg- ADD B (it adds the content of accumulator to the content of the register B)
7> Difference between Security and Protection ?
Ans: The main difference between security and protection lies within the fact that the security handles the external information threats in the computer systems whereas the protection deals with the internal threats.

Comments
Post a Comment